Course Introduction to Programming
First Program
In today's class, we will finally dive into the practical part and create our first program using VSCode, which we installed in the last class.
⚠️ This class is special because we are updating the course! Previously, we used the program CodeBlocks. You can check the instructions for using it in the video and at the end of the class.
File Creation
To start, we need to create a new file. To do this, first create a folder to store your codes and then open it in VSCode by clicking on "File" and Open Folder:
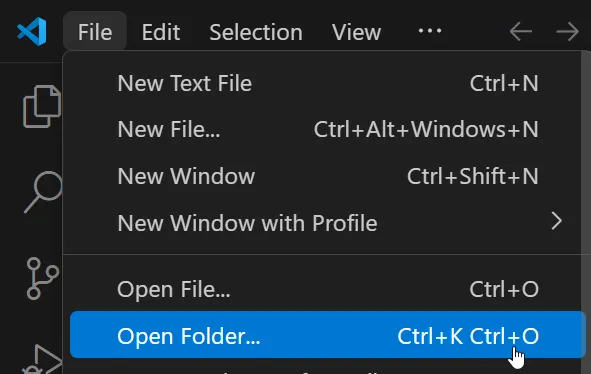
Figure 1
Select the folder on your computer that you want and click on "Select Folder".
If it asks if you trust the files, click "Yes".
Then, click on "New File" and type helloworld.c
:
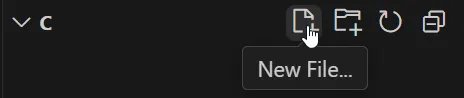
Figure 2
The file name ends with the
.c
extension. This is very important because it signals to the computer that the file in question contains code written in the C language.
Basic Structure and Compilation
In summary, a basic program in C consists of two parts: library inclusion and the main function.
Library Inclusion
Use the command #include <> to make the inclusion, passing the name of the module in question between the brackets. See:
#include <stdio.h>
These libraries are nothing more than a set of commands, codes, and functions already written that can be used by the programmer to facilitate and optimize their work routine.
Each library includes specific codes for a particular task. The one we used above has a name that means Standard Input-Output and we will use it whenever we need an interaction between the computer and the user.
Main Function
Called int main(), this function indicates where the program will start and which commands will be executed. These commands will be placed between the braces of main() and you will better understand this structure in the future. For now, focus on understanding and practicing. See:
#include <stdio.h> // Include the library
int main(){ // Declare the main function
// Code to be executed by the main function
}
Now, we can verify if our code is indeed written in C. So, let's run it. To do this, click on "Run Code" at the top of the screen:
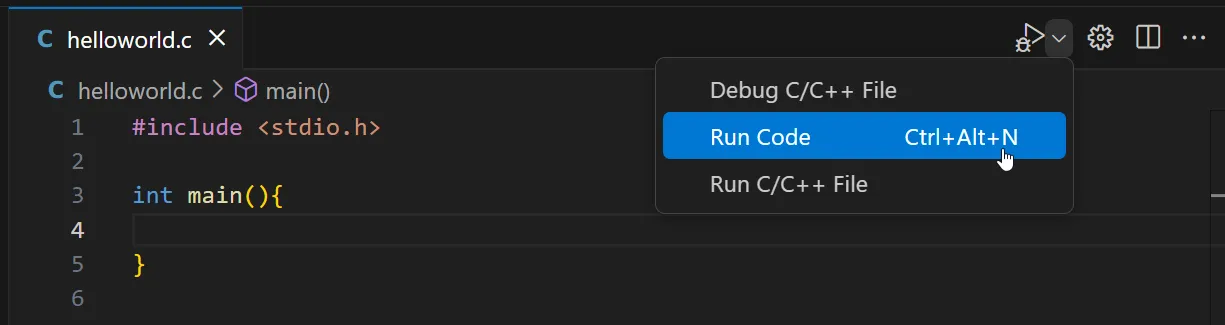
Figure 3
This button is a shortcut to compile and run the C file. The process is executed all at once when you click it.
A terminal will open and the necessary commands will be executed. Notice that the program in question does nothing.
Let's now provoke a compilation error to get an idea of how they are displayed. Let's change the function name main() to Main(), as we have seen, the C language is case sensitive. Note:
#include <stdio.h>
int Main(){
}
When trying to compile, the compiler does not find the main() function and we receive the error message:
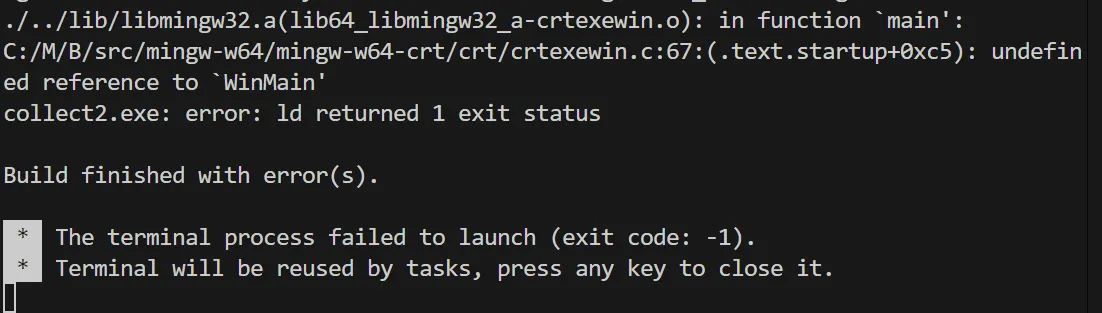
Figure 4
First Commands
printf() Command
Let's now see the basic commands for creating our first program. First, the printf() function, used to print data on the screen. You should pass what you want to print inside the parentheses and between double quotes. See in the example:
#include <stdio.h>
int main(){
printf("Ola Neps Academy");
}
After pressing to compile and execute, the program above prints the phrase Ola Neps Academy on the console (the black window we saw during execution).
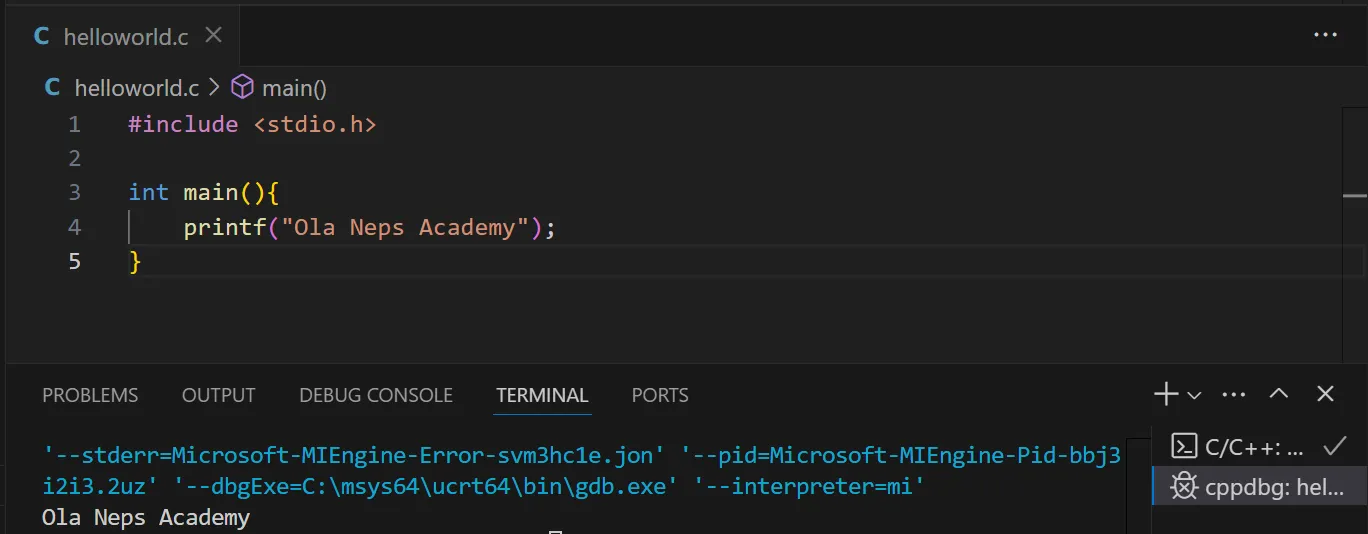
Figure 5
Note that at the end, a semicolon (***;***) was placed. Pay attention to this, as this ; is what delimits the end of the command and must always be placed at the end of each command.
Line Break
See the code below:
#include <stdio.h>
int main(){
printf("Ola Neps Academy");
printf("Ola Neps Academy");
}
When compiling and executing the program, we notice that even though we have placed two printf() commands on different lines, the actual print will be Ola Neps AcademyOla Neps Academy, on the same line. You should then use the special character \n where you want to make the break. Notice:
#include <stdio.h>
int main(){
printf("Ola Neps Academy\n");
printf("Ola Neps Academy\n");
}
Notice that now the print will be:
Ola Neps Academy
Ola Neps Academy
Note that it is also possible to break within the same printf(), just pass the character in the middle of the phrase. Observe:
#include <stdio.h>
int main(){
printf("Ola\nNeps Academy\n");
printf("Ola Neps Academy\n");
}
The print will be:
Ola
Neps Academy
Ola Neps Academy
For now, we will stop here. We have learned about file creation, the basic structure of a program in C, and the first command, printf(). It is emphasized again the importance of remembering to place the semicolons, as most compilation errors result from their absence.
See you next time! Happy studying!
Using Code Blocks
This section belongs to the old version of the course. If you do not want to use VSCode, follow the steps below.
To create a file, we have two options:
First method (simplest):
In the upper left corner of the screen, go to File >> New >> Empty file (or simply Ctrl + Shift + N) to create the file.
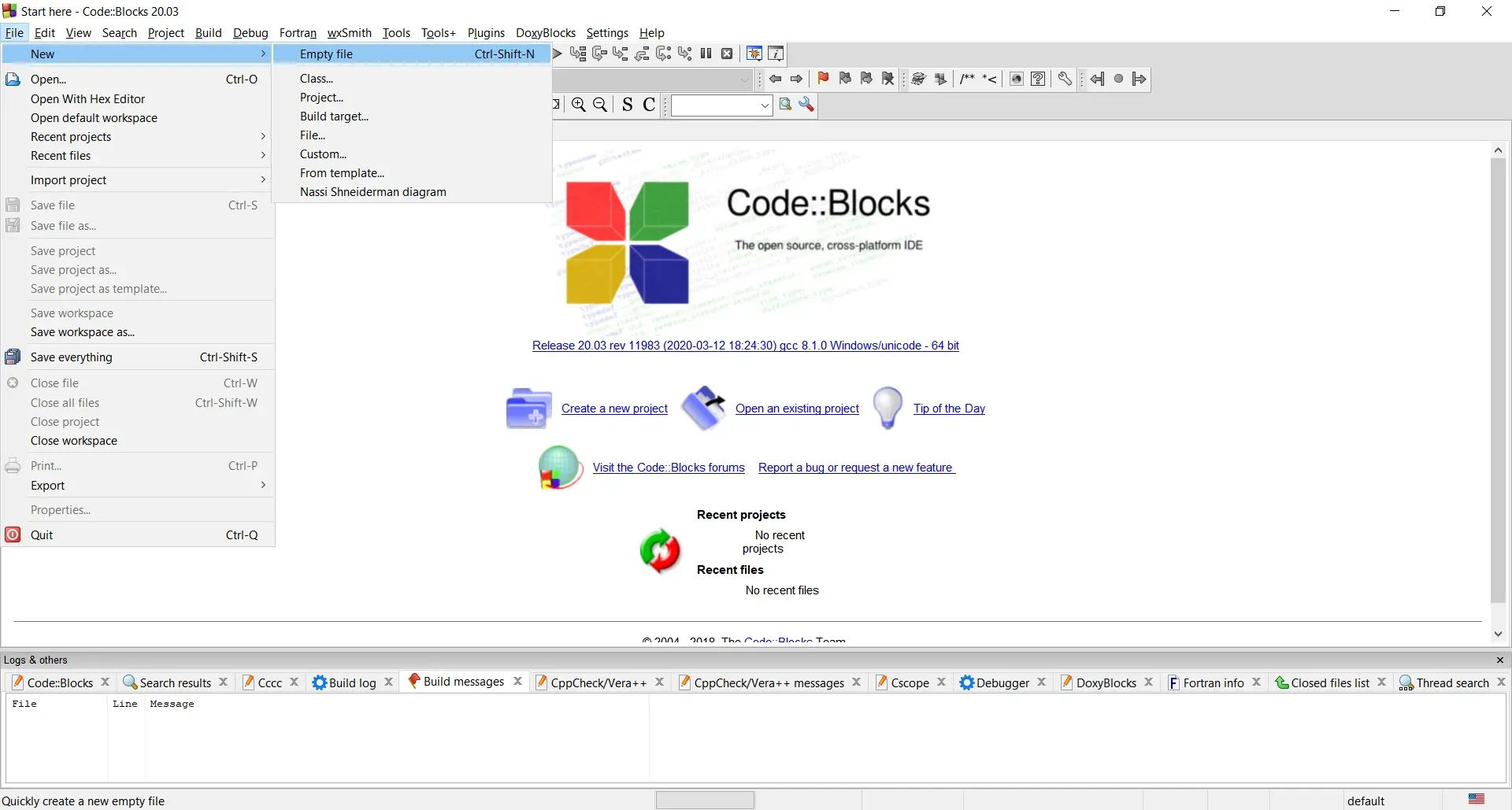
Figure 6
Go to File >> Save file (or simply Ctrl + S) to open the window to save the file.
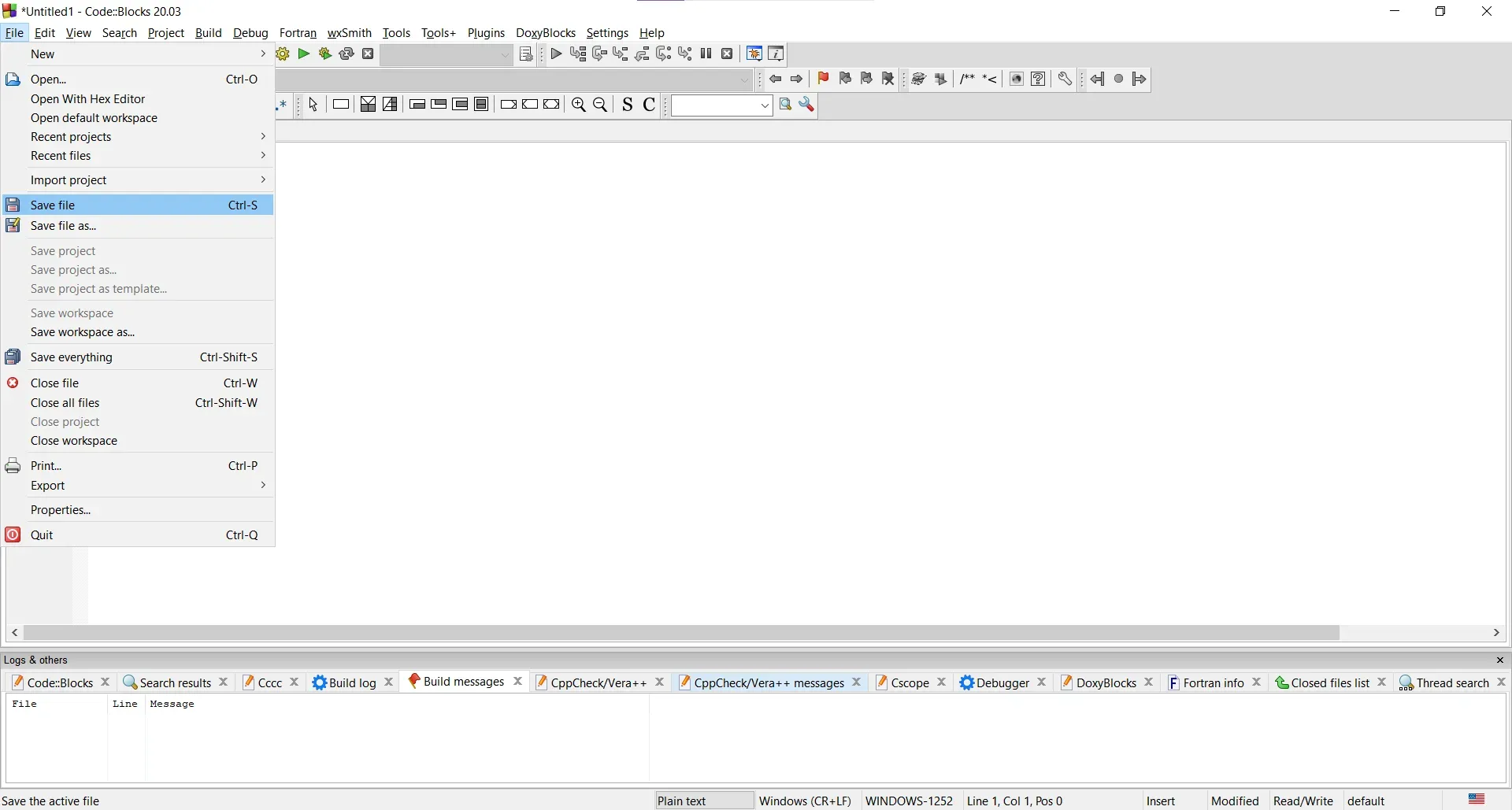
Figure 7
Choose a name of your preference and end with the extension .c. This is very important as it signals to the computer that the file in question contains code written in the C language. Press Save to save.
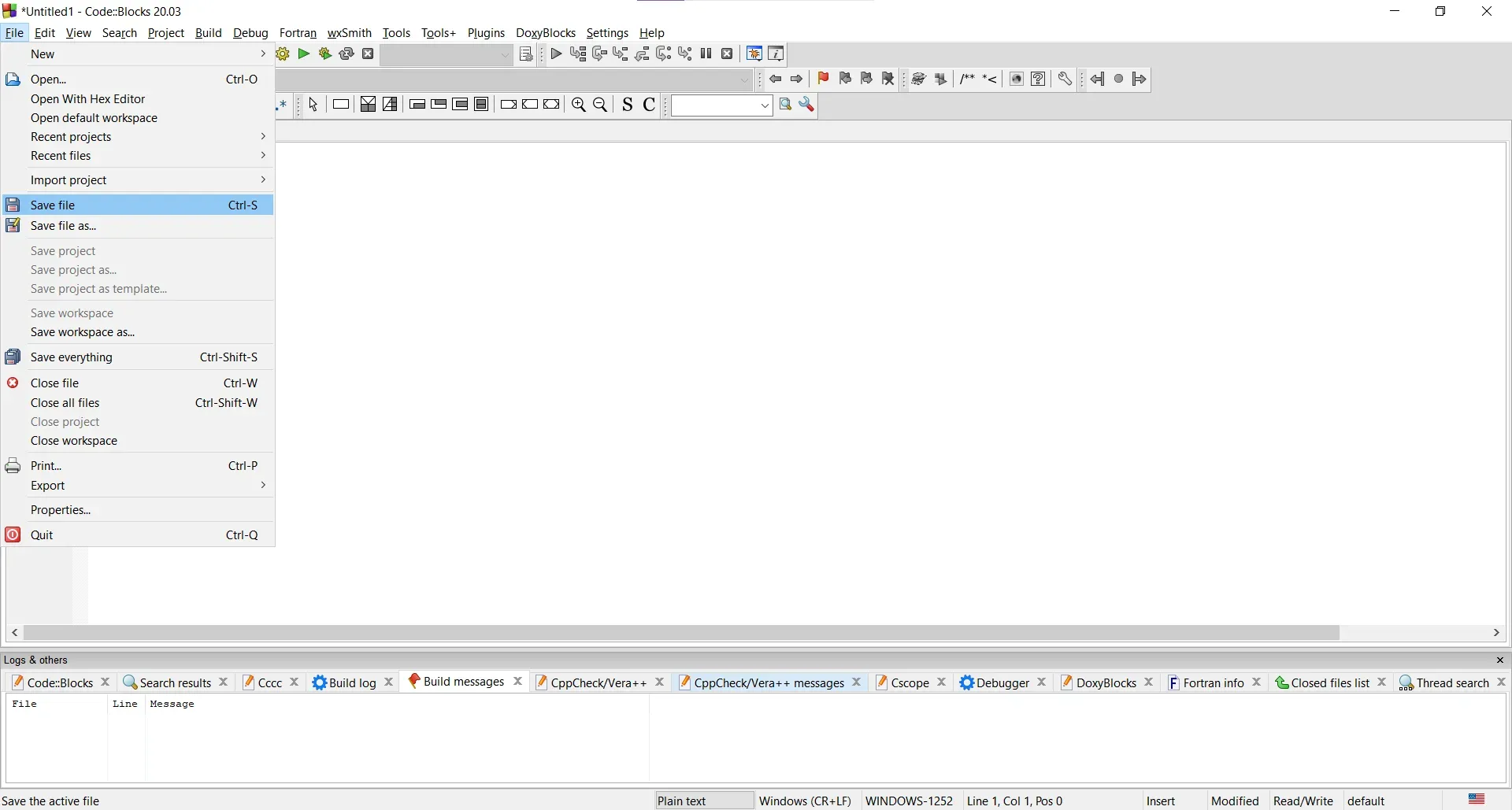
Figure 8
Second method:
In the upper left corner of the screen, go to File >> New >> File....
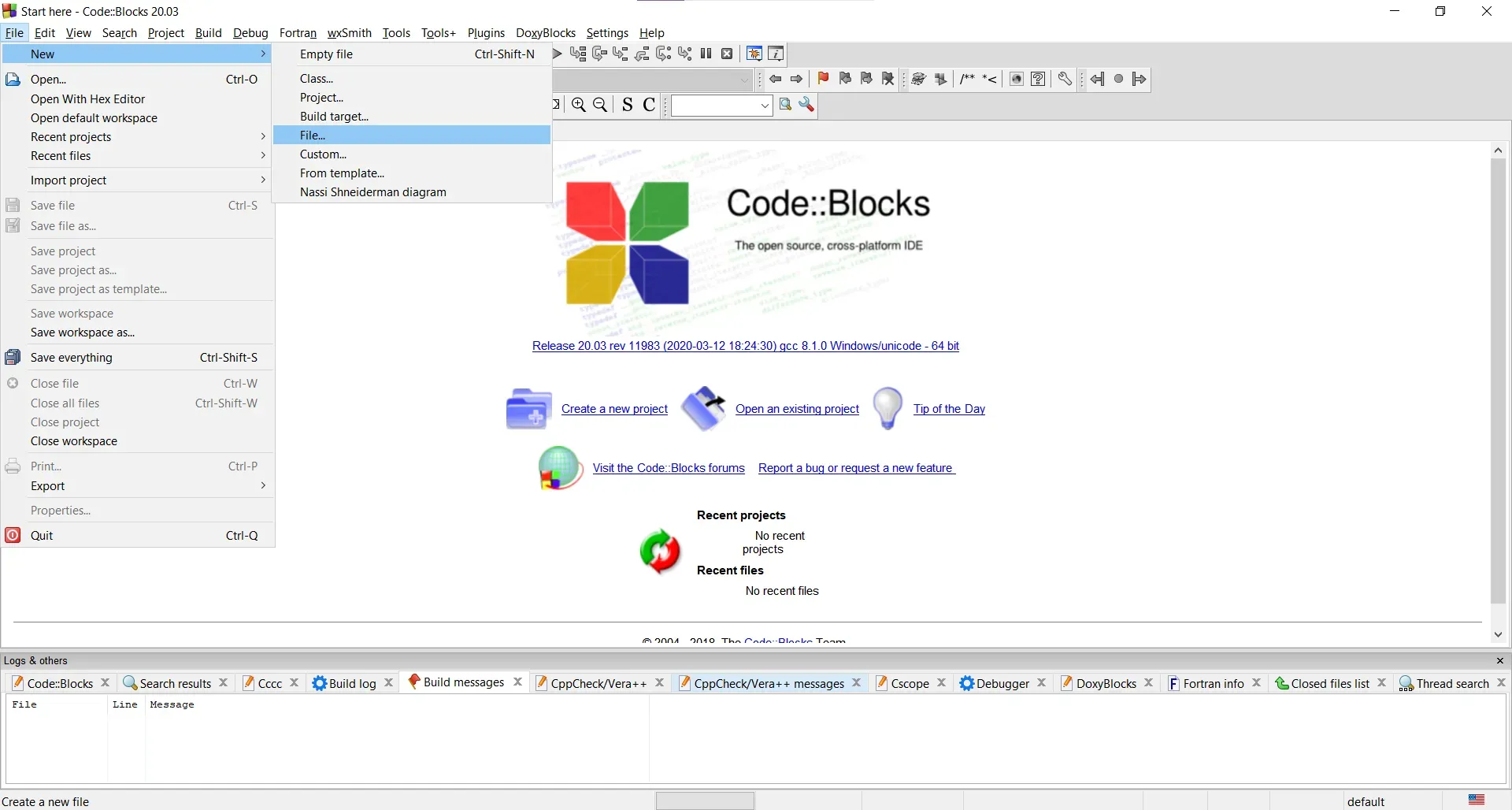
Figure 9
Click on C/C++ Source and then Go.
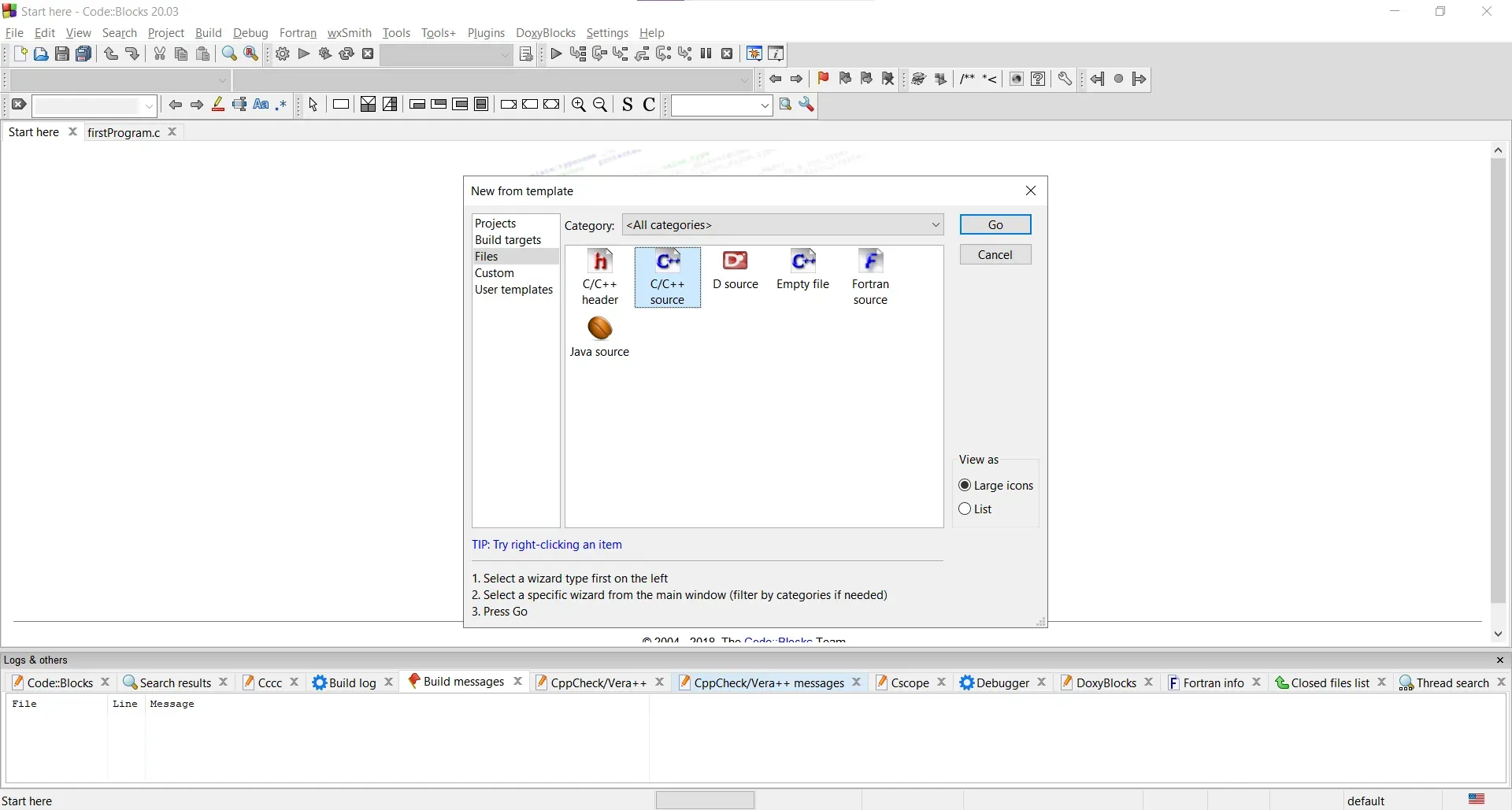
Figure 10
Check the box next to Skip this page next time and then Next (this only the first time you create a file so that this window does not show again).
Choose the language in question (in our case, C) and click Next.
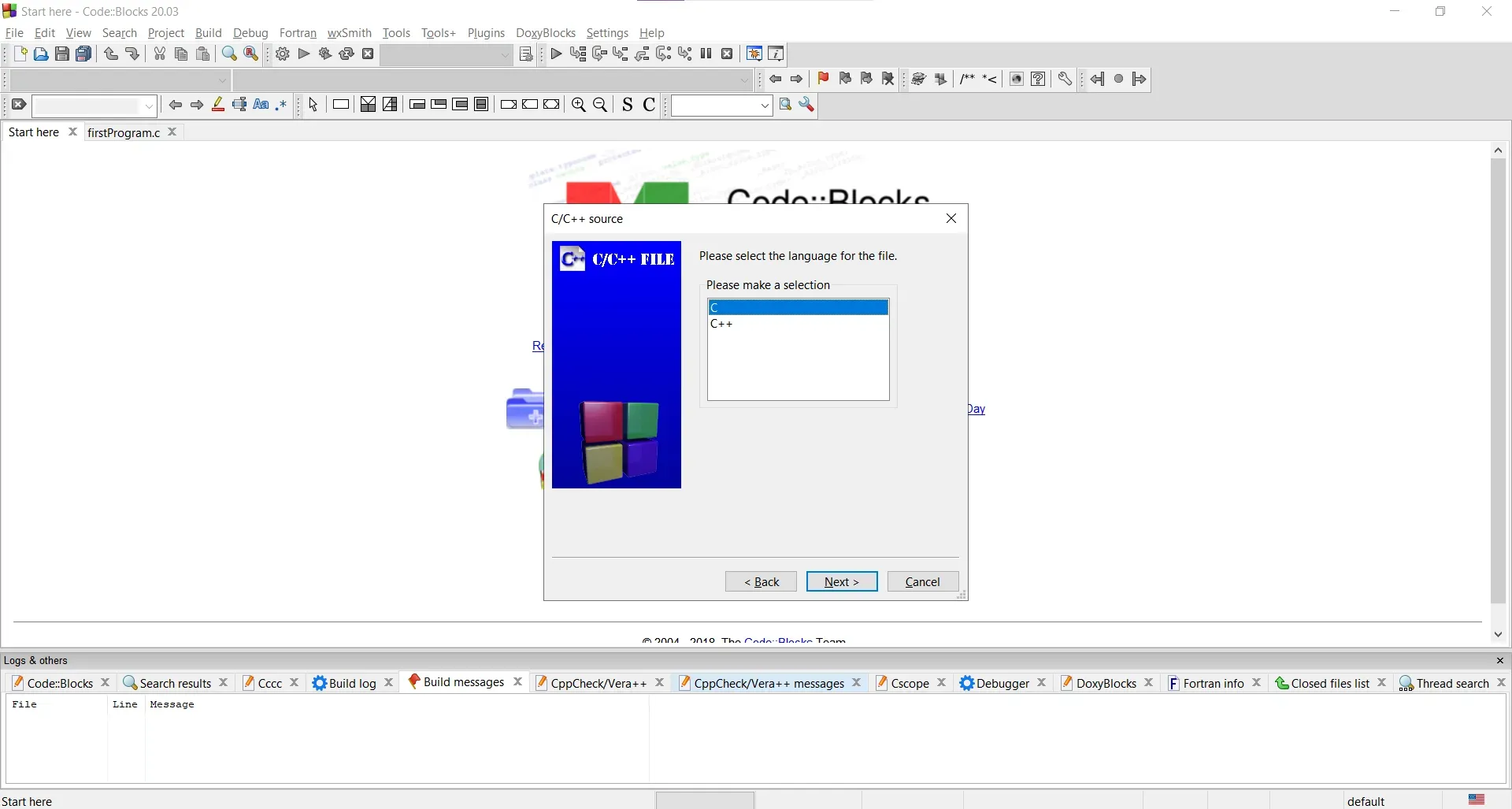
Figure 11
Press the box with ellipses.
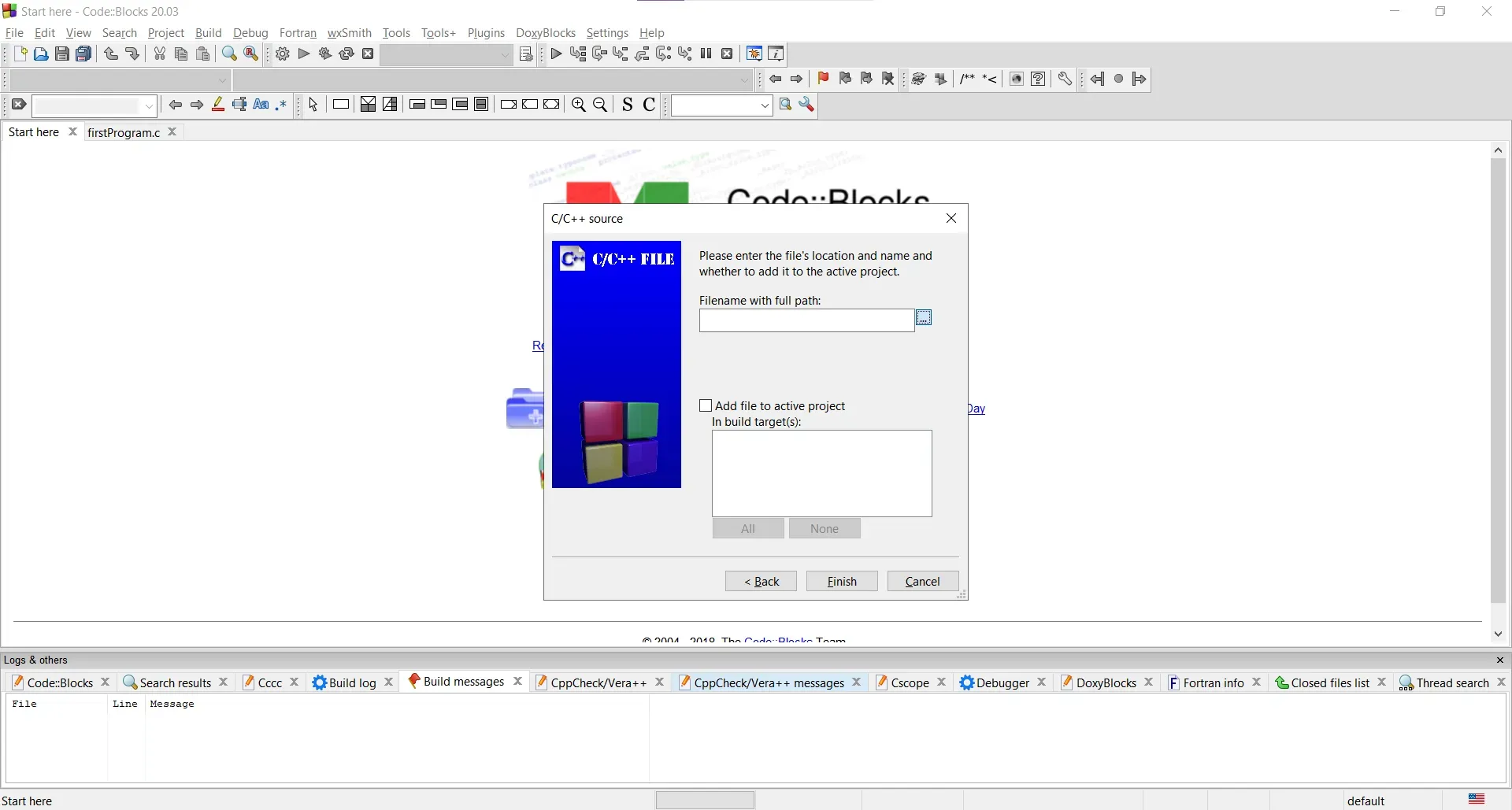
Figure 12
Go to the location where you want to save the file and write a name of your preference next to File name:. Now, there is no need to specify the extension because this has already been done previously.
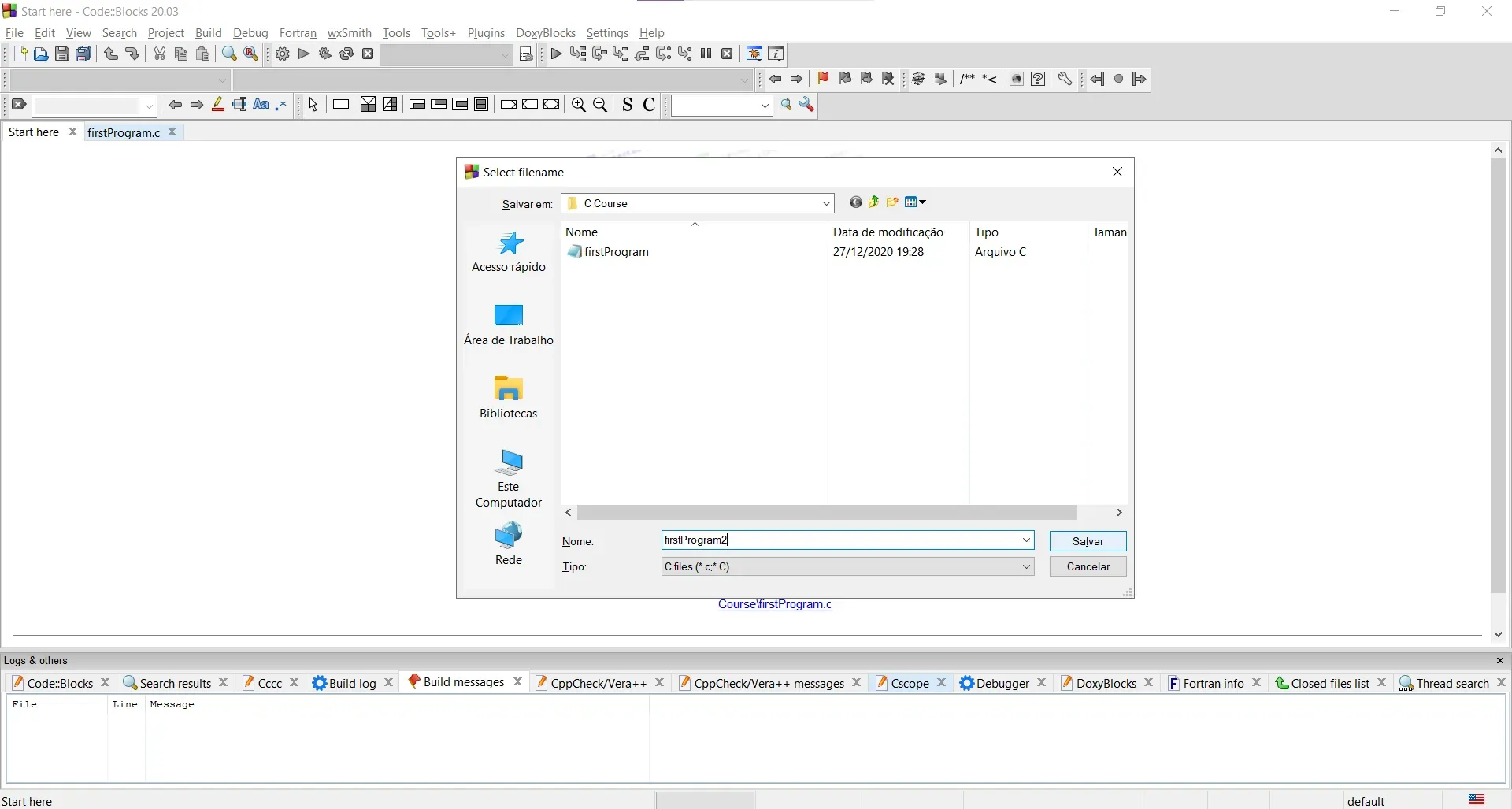
Figure 13
Click Finish to conclude.
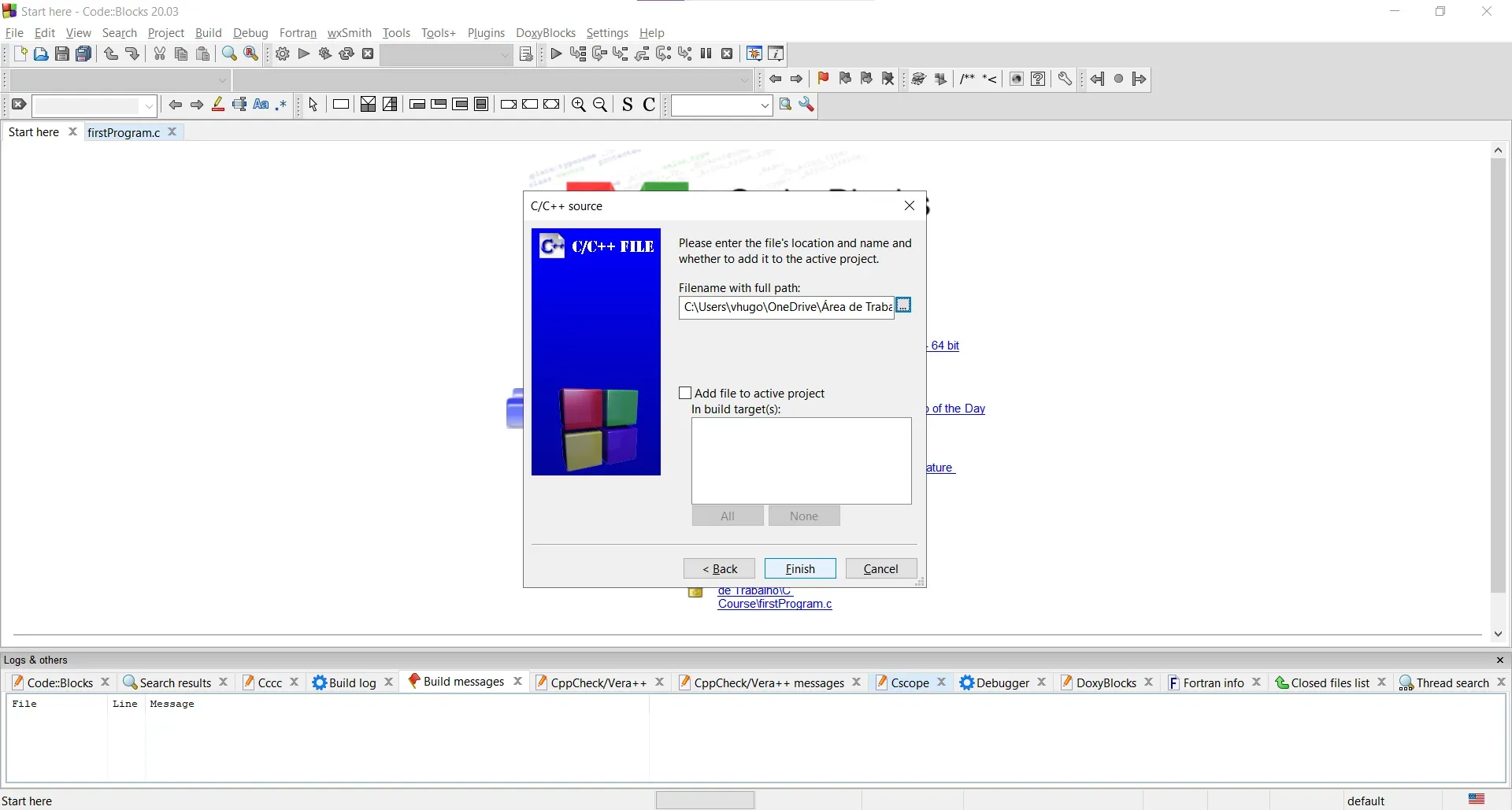
Figure 14
Main function
As the first code, use:
#include <stdio.h> // Includes the library
int main(){ // Declares the main function
// Code to be executed by the main function
}
To verify if our code really is a C code, let's compile it: for this, in Code::Blocks, click on the gear at the top of the screen. Whether there is an error or not, the compiler will point it out and Code::Blocks will show it in the Build Messages tab.
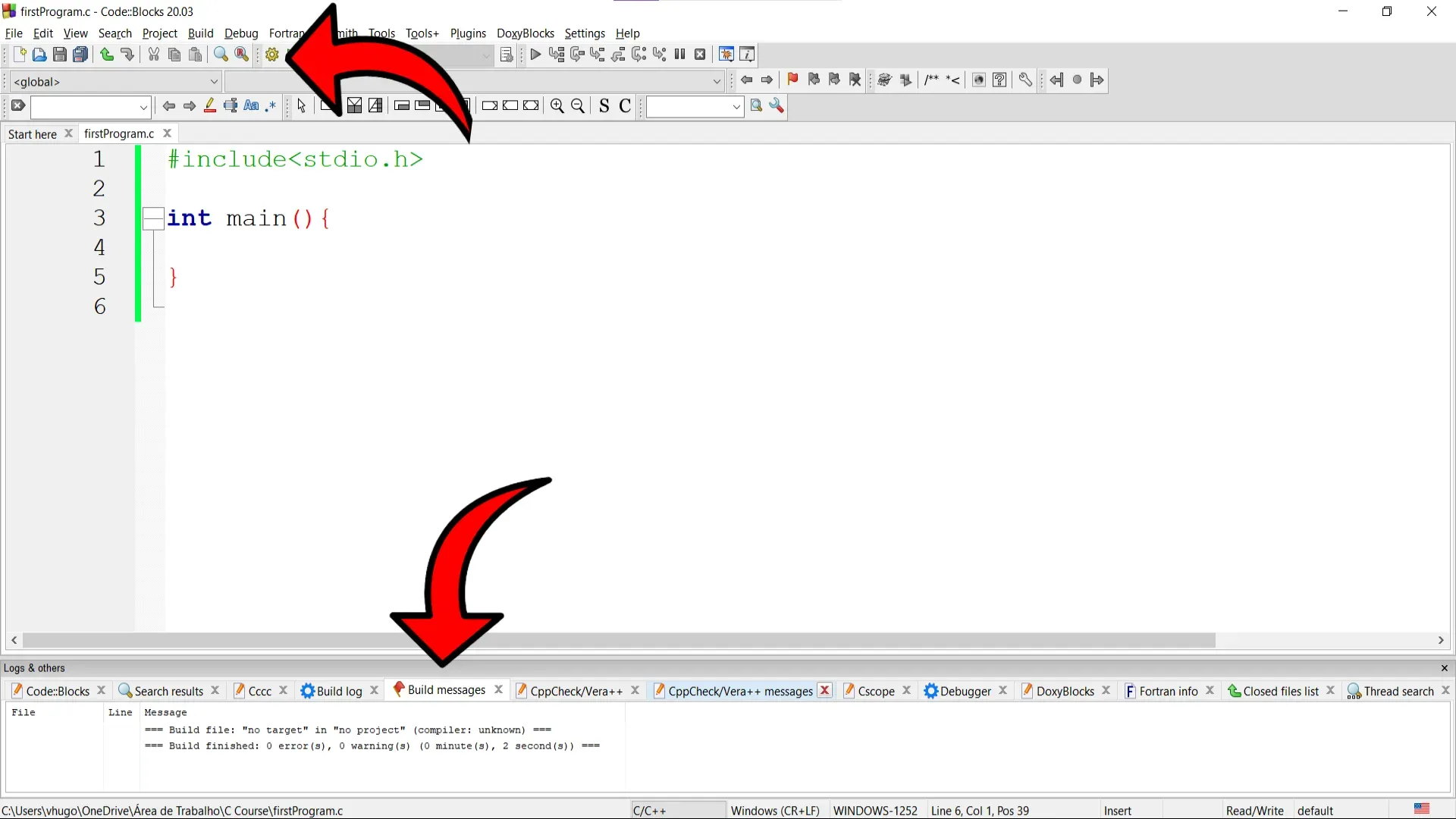
Figure 15
The program in question does nothing. You can verify this by executing it through the button with a green arrow next to the gear we just used to compile.
To provoke a compilation error, let's change the name of the function main() to Main():
#include <stdio.h>
int Main(){
}
When we try to compile, the compiler does not find the function main() and we receive the error message:
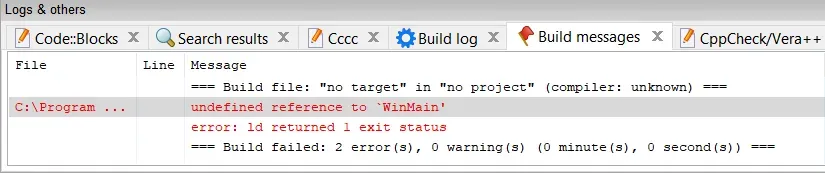
Figure 16
Compilation and execution warning
Let's say we compiled and executed the code below:
#include <stdio.h>
int main(){
printf("Ola Neps Academy");
}
If we change the code a bit, modifying what will be printed:
#include <stdio.h>
int main(){
printf("Ola de novo Neps Academy");
}
If you just press to run, notice that Ola Neps Academy from the previous program will be printed again. This happens because for each change in the code, we need to perform the compilation again.